How to create a new custom Woocommerce Payment Gateways with Woocommerce Checkout Blocks support
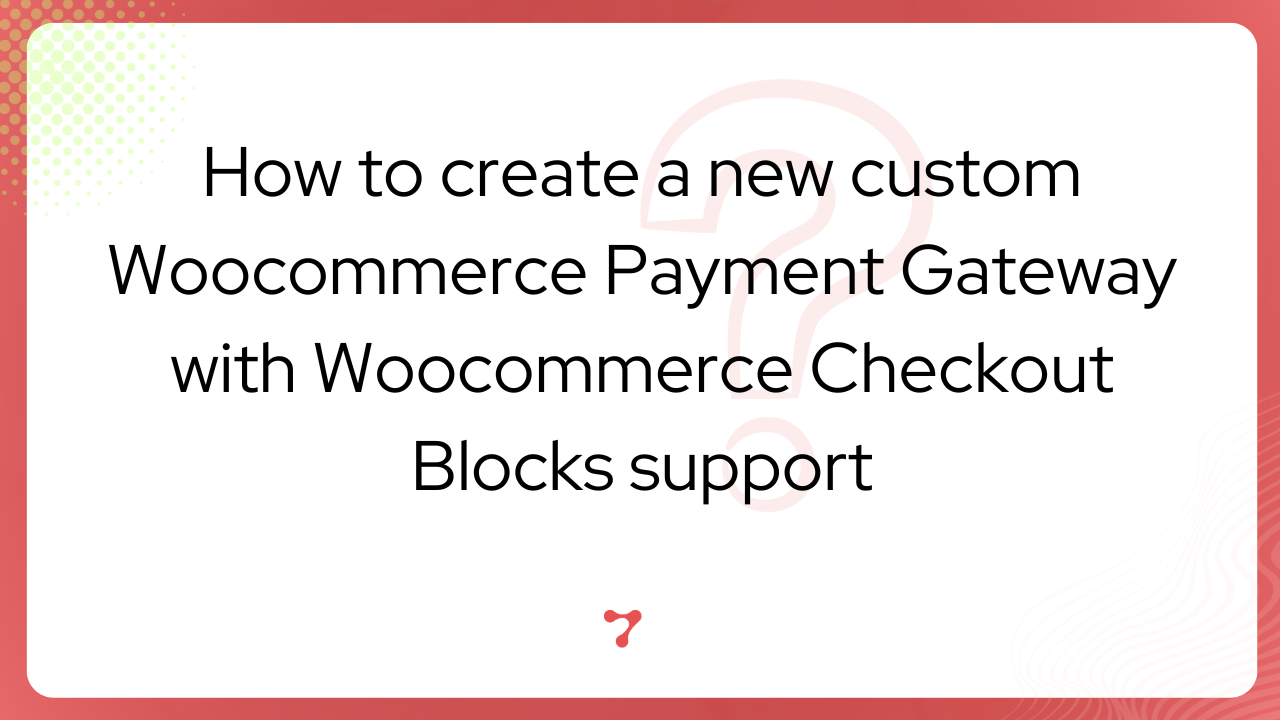
Are you a custom woocommerce developer? Are you facing any issues with creating a woocommerce cart and checkout blocks? In today’s article, I can certainly guide you on how to create a new custom WooCommerce payment gateway with support for WooCommerce checkout blocks. WooCommerce, the popular WordPress plugin, offers a wide range of payment gateways out of the box. However, there are times when you need a custom solution to meet specific business requirements. In this tutorial, we will guide you through the process of creating a new custom payment gateway for WooCommerce checkout blocks, named “My Custom Gateway”.

Step 1: Create a plugin
To enable a woocommerce custom woocommerce payment gateway, first, develop a plugin. This plugin will serve to activate and integrate the specified custom payment gateway seamlessly into the system.
/* Plugin Name: Custom Gateway Description: A custom payment gateway for WooCommerce. Version: 1.0.0 Author: Dev team Sevengits Author URI: sevengits.com License: GPL-2.0+ License URI: http://www.gnu.org/licenses/gpl-2.0.txt Text Domain: custom-gateway Domain Path: /languages */
Step 2: Create the Gateway Class
Inside the “my-custom-gateway” folder, create a PHP file (e.g., class-gateway.php) and define your custom gateway class. This class should extend the WC_Payment_Gateway
class provided by WooCommerce.
<?php class My_Custom_Gateway extends WC_Payment_Gateway { // Constructor method public function __construct() { $this->id = 'my_custom_gateway'; $this->method_title = __('My Custom Gateway', 'my-custom-gateway'); $this->method_description = __('Accept payments through My Custom Gateway', 'my-custom-gateway'); // Other initialization code goes here $this->init_form_fields(); $this->init_settings(); add_action('woocommerce_update_options_payment_gateways_' . $this->id, array($this, 'process_admin_options')); } public function init_form_fields() { $this->form_fields = array( 'enabled' => array( 'title' => __('Enable/Disable', 'my-custom-gateway'), 'type' => 'checkbox', 'label' => __('Enable My Custom Gateway', 'my-custom-gateway'), 'default' => 'yes', ), // Add more settings fields as needed ); } // Process the payment public function process_payment($order_id) { $order = wc_get_order($order_id); // Implement your payment processing logic here // Mark the order as processed $order->payment_complete(); // Redirect to the thank you page return array( 'result' => 'success', 'redirect' => $this->get_return_url($order), ); } } ?>
Step 3: Hook into WooCommerce
In your main plugin file hook into WooCommerce to add your custom gateway. In the main plugin file, you can hook into WooCommerce to seamlessly integrate your custom gateway. This involves utilizing the appropriate hooks and actions provided by the WooCommerce framework to ensure smooth integration. By implementing this connection, your custom gateway becomes an integral part of the WooCommerce payment options.
<?php add_action('plugins_loaded', 'woocommerce_myplugin', 0); function woocommerce_myplugin(){ if (!class_exists('WC_Payment_Gateway')) return; // if the WC payment gateway class include(plugin_dir_path(__FILE__) . 'class-gateway.php'); } add_filter('woocommerce_payment_gateways', 'add_my_custom_gateway'); function add_my_custom_gateway($gateways) { $gateways[] = 'My_Custom_Gateway'; return $gateways; } ?>
Adding Checkout block support
Step 1: Declare the plugin support WooCommerce Checkout Blocks.
Users can now experience enhanced convenience and efficiency during the checkout phase with the declared plugin. Add this to the plugin’s main file.
/** * Custom function to declare compatibility with cart_checkout_blocks feature */ function declare_cart_checkout_blocks_compatibility() { // Check if the required class exists if (class_exists('\Automattic\WooCommerce\Utilities\FeaturesUtil')) { // Declare compatibility for 'cart_checkout_blocks' \Automattic\WooCommerce\Utilities\FeaturesUtil::declare_compatibility('cart_checkout_blocks', __FILE__, true); } } // Hook the custom function to the 'before_woocommerce_init' action add_action('before_woocommerce_init', 'declare_cart_checkout_blocks_compatibility');
Step 2: Register WooCommerce Checkout Blocks payment method type registration.
To register the WooCommerce Checkout Blocks payment method type, initiate the registration process to seamlessly integrate it with the platform. This ensures compatibility and accessibility for users seeking diverse payment options within the WooCommerce ecosystem.
// Hook the custom function to the 'woocommerce_blocks_loaded' action add_action( 'woocommerce_blocks_loaded', 'oawoo_register_order_approval_payment_method_type' ); /** * Custom function to register a payment method type */ function oawoo_register_order_approval_payment_method_type() { // Check if the required class exists if ( ! class_exists( 'Automattic\WooCommerce\Blocks\Payments\Integrations\AbstractPaymentMethodType' ) ) { return; } // Include the custom Blocks Checkout class require_once plugin_dir_path(__FILE__) . 'class-block.php'; // Hook the registration function to the 'woocommerce_blocks_payment_method_type_registration' action add_action( 'woocommerce_blocks_payment_method_type_registration', function( Automattic\WooCommerce\Blocks\Payments\PaymentMethodRegistry $payment_method_registry ) { // Register an instance of My_Custom_Gateway_Blocks $payment_method_registry->register( new My_Custom_Gateway_Blocks ); } ); }
Step 3: Create a checkout block-based payment gateway class
Develop a woocommerce checkout block-based payment gateway class to facilitate seamless integration and interaction with modern checkout block-based systems. This class enables efficient management and processing of payments within the context of evolving woocommerce checkout block-based technologies.
<?php use Automattic\WooCommerce\Blocks\Payments\Integrations\AbstractPaymentMethodType; final class My_Custom_Gateway_Blocks extends AbstractPaymentMethodType { private $gateway; protected $name = 'my_custom_gateway';// your payment gateway name public function initialize() { $this->settings = get_option( 'woocommerce_my_custom_gateway_settings', [] ); $this->gateway = new My_Custom_Gateway(); } public function is_active() { return $this->gateway->is_available(); } public function get_payment_method_script_handles() { wp_register_script( 'my_custom_gateway-blocks-integration', plugin_dir_url(__FILE__) . 'checkout.js', [ 'wc-blocks-registry', 'wc-settings', 'wp-element', 'wp-html-entities', 'wp-i18n', ], null, true ); if( function_exists( 'wp_set_script_translations' ) ) { wp_set_script_translations( 'my_custom_gateway-blocks-integration'); } return [ 'my_custom_gateway-blocks-integration' ]; } public function get_payment_method_data() { return [ 'title' => $this->gateway->title, //'description' => $this->gateway->description, ]; } } ?>
Step 4: Create js files for the block.
Generate JavaScript files for the block to implement and enhance its functionality within the designated application. These files encapsulate the necessary code and logic required to empower the checkout block’s dynamic behavior and user interactions.
const settings = window.wc.wcSettings.getSetting( 'my_custom_gateway_data', {} ); const label = window.wp.htmlEntities.decodeEntities( settings.title ) || window.wp.i18n.__( 'My Custom Gateway', 'my_custom_gateway' ); const Content = () => { return window.wp.htmlEntities.decodeEntities( settings.description || '' ); }; const Block_Gateway = { name: 'my_custom_gateway', label: label, content: Object( window.wp.element.createElement )( Content, null ), edit: Object( window.wp.element.createElement )( Content, null ), canMakePayment: () => true, ariaLabel: label, supports: { features: settings.supports, }, }; window.wc.wcBlocksRegistry.registerPaymentMethod( Block_Gateway );
Screenshots
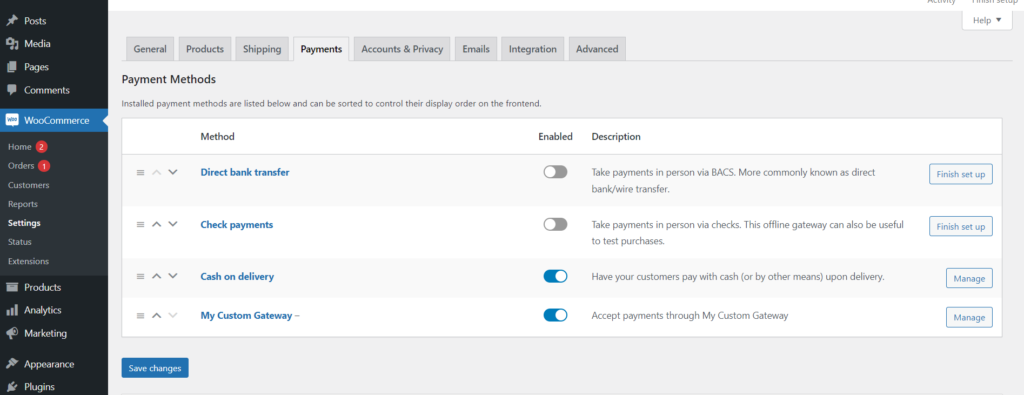
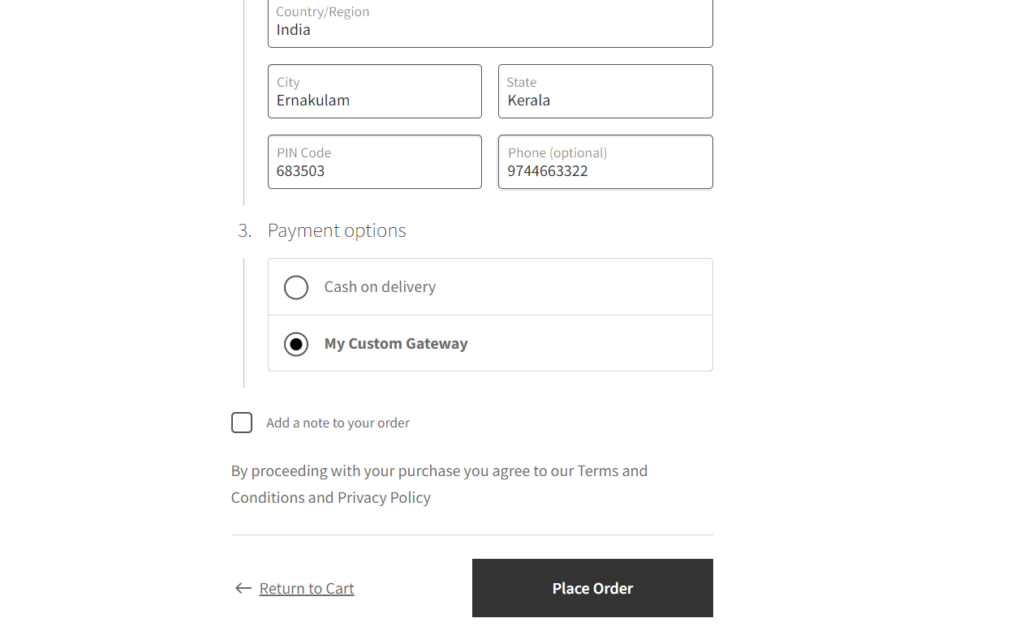
To download the zip folder use the below link :
Conclusion
In today’s article, I can certainly guide you on how to create a new custom WooCommerce checkout payment gateway with support for WooCommerce checkout blocks. However, there are times when you need a custom solution to meet specific business requirements.
If you have any queries about Integrating Custom Payment Gateways with WooCommerce Checkout Blocks – learn more
If you’re searching for a reliable location picker plugin, we strongly endorse the WooCommerce Checkout Location Picker. Get it now!
If you’re interested in a WooCommerce WhatsApp plugin with support for WooCommerce checkout blocks, take a look now!
If you have more queries about Woocommerce Cart and Checkout Blocks- Click Here
Require assistance developing a custom WooCommerce payment plugin? Let’s chat and create a specialized solution for your needs.
I am encountering an issue with the WooCommerce checkout block. I successfully displayed my custom payment gateway on the WooCommerce checkout block, but the fields are not showing after selecting my payment gateway.
Hello jahagit,
The Blocked Checkout feature is a recent addition to WooCommerce; however, there is a limited availability of tutorials or code snippets addressing this specific functionality. Given that many developers come from a PHP background, customizing checkout fields now demands familiarity with React. Unfortunately, I lack experience in React development, making it challenging for me to provide guidance on adding fields to the checkout page without resorting to React coding.
I encourage fellow developers to share any resources or links related to customizing the checkout page without relying on React. This would greatly benefit those who, like me, are not well-versed in React development. At present, the most comprehensive tutorial for Blocked Checkout is being referenced by numerous developers, and any additional information shared here could prove invaluable to the community
Dear team!
Thank you so much for this guide.
But i have an issue, i active one custom payment gateway method then i create more another custom payment method. I can’t active it cause my site throw the error: “Plugin could not be activated because it triggered a fatal error.” I can’t enable those 2 plugins together. Could you help me to resolve this issue? Thank you guys.
//WP: version 6.4.1
//WC: version 8.5.2
//PHP: version 8
Because you have more than 1 function named like ‘woocommerce_myplugin’
PHP Fatal error: Cannot redeclare woocommerce_myplugin()
I cannot display the name of my payment gateway it always shows default label
And now there’s fatal error when two payments plugins are there
I duplicate ‘Custom gateway’ to ‘Custom gateway 2’ and change all ‘my_custom_gateway’ to ‘my_custom_gateway2’, the my_custom_gateway2 is OK, but the original my_custom_gateway will be incompatible.